Data Structure -1 (Arrays) Class 12 Computer Science
Data Structure -1 (Arrays)
Class 12 Computer Science
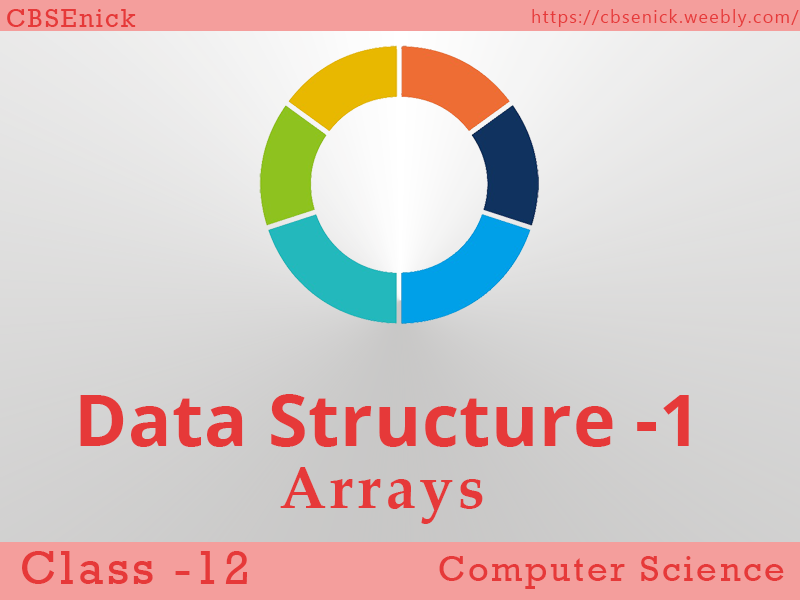
1. Write a program to find the saddle points in a matrix. It is computed as follows:
- Find out the smallest element in a row. The saddle point exists in a row if an element is the largest element in that corresponding column. For instance, consider the following matrix
10 2 3
1 3 3
- The saddle point results are listed below:
b) In row 2, saddle point does not exist.
c) In row 3, saddle point does not exist.
|
2. Write a user defined function in C++ to find and display the row sums of two-dimensional array.
#include<iostream.h>
#include<conio.h>
void main()
{
clrscr();
int a[3][3];
int i,j,s=0,sum=0;
cout<<"Enter 9 elements of 3*3 Matrix \n";
for(i=0;i<3;i++)
for(j=0;j<3;j++)
cin>>a[i][j];
cout<<"Matrix Entered By you is \n";
for(i=0;i<3;i++)
{for(j=0;j<3;j++)
cout<<a[i][j]<<" ";
cout<<endl;
}
for(i=0;i<3;i++)
{for(j=0;j<3;j++)
s=s+a[i][j];
cout<<"sum of row "<<i+1<<" is "<<s;
s=0;
cout<<endl;
}
getch();
}
Comments
Post a Comment